Testing React Native With the New Jest - Part II
It’s a well known paradigm in the React world for any big app you want to write to extract your business logic (aka manage the state of your app) out of your components. The majority of your components should be a small piece of reusable code that just renders UI based on the app state that is triggered mainly by: User input and API calls. The same paradigm is used in React Native.
I will talk about Redux together with React Native since testing Redux is easy. However feel free to apply the same concepts to another state management you choose. That’s what we will do here:
- Testing Reducers: Manual comparison vs. Snapshots
- Mock the built-in React Native fetch
- Re-mock the redux-mock-store
- Testing Actions (including async ones): Manual comparison vs. Snapshots
Please refer to Unit testing React Native with the *new* Jest (I) for setting up Jest with React Native.
Reducers
Testing a reducer might be one of the easiest parts to test from your app: Given the current state and an action, a reducer should return a new state after that action is applied. Let’s see how to test part of this example:
We will focus on testing that LOAD_REPOS.success action. Let’s create version 1 of our test:
Copy & paste again :(
Quite short, right? I just went and copied and pasted the initialStatefrom my reducer and added the part that changes (repos). When you work in larger projects and your state starts to grow (0r you do some refactoring), you will find this process to be so repetitive that you will want to do something better, so, version 2:
Reimplementing the reducer :(
This is better, right? I can add or delete anything to the state (except the isLoading & repos part) and I won’t break the test. I just need to go back here if that action.success does actually affect another part of the state in the future. But, don’t you feel that test it is actually very similar to:
Again, what we are doing in the test is basically reimplementing the reducer. Anytime I change the logic in my reducer, I need to come back here and do the same. It get’s so repetitive… Can we do better? Let’s snapshot that output. Hello version 3:
Given the state and an action, generate the output for me
I know, it’s just one line and I did not have to repeat myself at all (DRY?). Do you like it? I do! Let’s see what the snapshot looks like:
Wow! I can actually see how my state will exactly look like after applying the action. So as you can see, we are forcing Jest to do the hard work while we are just watching. In this case, if you add or remove any part of your state, this test will break: Which is what you should expect, since the outputted state would be different. But as I explained in the first part of these series, the only thing we need to do here is:
- Review the Jest error output
- If it’s expected, run the test again using -u to re-generate the snapshot:
- If it’s not expected, go and fix it (obviously)
This is a great example of how fast and easy is to write your tests first, aka TDD (Test-driven development):
- Write your test first
- Start writing your reducer with the Jest — watch mode enabled
- Once the outputted snapshot is the one you are expecting, just tell Jest to save it
- Done!
Actions
A simple action creator is just a function that returns an object:
Simple action creator
Which can be tested like:
For the second part, let’s check out the generated snapshot:
Yea, the exact same thing as the reducer: I (and my team) can read the output instead of re-creating the action again (which includes the need to maintain that code in both places).
By the way, if you are already bored of reading, you can watch the same concept in a nice and short explanation thanks to Kent C. Dodds. If you are not, let’s continue. Since testing such simple actions is so meh, let’s go and test an async action that uses the built-in fetch of React Native and dispatches more than one action at once.
Async actions
This is the async action we will test:
We need some mocking help here to be able to have a nice way to test that so… Let’s mock!
Mocking the built-in fetch of React Native
Similar way that you can use whatwg-fetch in your web apps which will include a fetch polyfill into window.fetch, React Native uses the same idea and includes fetch into the global object as global.fetch.
We don’t want to make real api calls into our unit tests but we would like to mock the responses to write better assertions. There are some mock libraries out there but I just wrote a smaller version that fits my purposes:
Mocking fetch
Then, we can tell Jest to load that config using the package.json field setupFiles:
You will see when we are testing our async action how I used this mock. For now, let’s continue mocking.
Mocking a Redux store
I am sure that a lot of Redux users are familiar with redux-mock-store. It’s a simple helper that provides a mocked version of the Redux store and makes it very easy to test what actions have been called. Once you have your mocked store, you can use store.dispatch and get the list of actions that has been dispatched using store.getActions.
But you know what? I feel so lazy that I don’t want to do the same in all my tests where I need a store and a thunk, so I am going to re-mock the redux-mock-store module. See, in Jest you can create a __mocks__folder in the same level as your node_modules to mock any npm module. So I can actually create a redux-mock-store.js file (name of the module) in the __mocks__ folder:
And use it in my tests like:
Lazy, eh?
Async test
I feel that we are ready to test our async action now, don’t you? Basically what we want to test here is:
- A successful request should trigger request & success actions
- A failure request should trigger request & failure actions
First case:
Rather short, right?
And what output will be generated?
Just as expected and in this case, I think this one is very useful and easy to read: Quick read by reading “type” and longer read by checking that actually the “data” matches what we told fetch to return.
The second case is very similar:
And its output:
As you can see, when you have the mocks in place, writing this kind of tests become very straightforward, and most likely, you won’t need to mock anything since you can find them out there or write your own and reuse them for other projects.
Wrap up
This is it, with this part and the first one, we pretty much covered (I hope) unit testing of your React Native components + your state management logic. We also re-discovered Jest and its new superpower: Snapshots. On contrary, I am sure the way to test React Native apps will continue to improve as much as Jest keeps growing. So this article might get out of date quite soon!
Let me finish with some personal thoughts:
Why would I use Jest?
- You can easily test your React & React Native components
- Snapshots are a plus, but they are totally optional
- You can easily test Redux or other state management libraries with Jest: Shared logic between web and mobile could share tests too!
- The same community have created React Native & Jest. What does that mean? They will be the ones who care the most about making React Native and Jest to play nicely together in the future
To sum up: If you test your web & mobile app with Jest, you can use the same core principle of React Native:
Learn once, write anywhere — React Native
Of course, that is only my opinion. I just hope you enjoyed this short journey about testing a React Native app with the new Jest and remember… Happy testing!
You can also check our services offered by our React Native development company.
Learn more about
Testing
Here's everything we published recently on this topic.
We can help you move
it forward!
At Callstack, we work with companies big and small, pushing React Native everyday.
Quality Assurance
Combine automated and manual testing with CI/CD integration to catch issues early and deliver reliable React Native releases.
Release Process Optimization
Ship faster with optimized CI/CD pipelines, automated deployments, and scalable release workflows for React Native apps.
React Compiler Implementation
Use React Compiler to achieve instant performance benefits in your existing applications.
React Native Trainings
Equip your team with React Native skills through tailored training sessions.
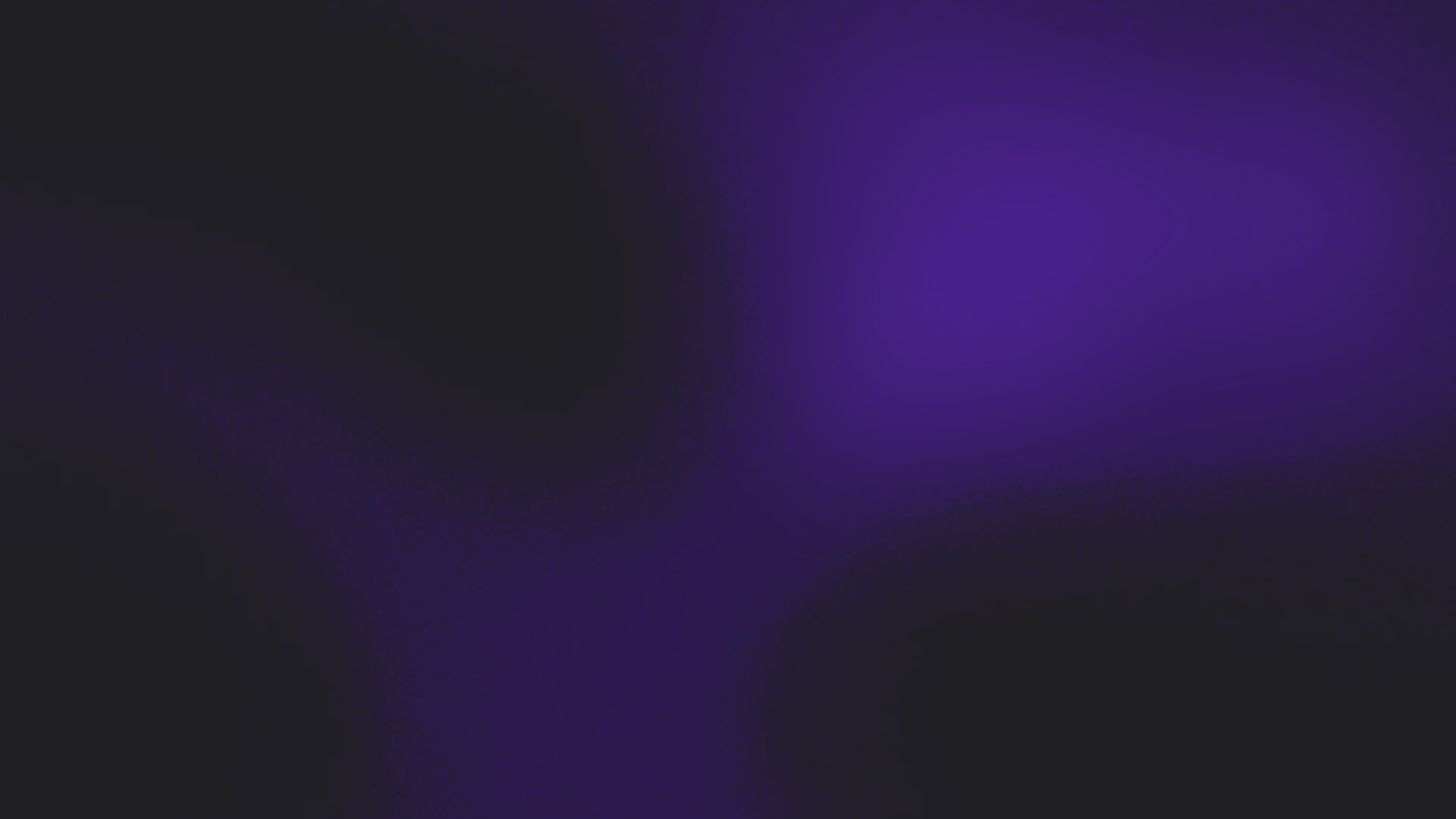